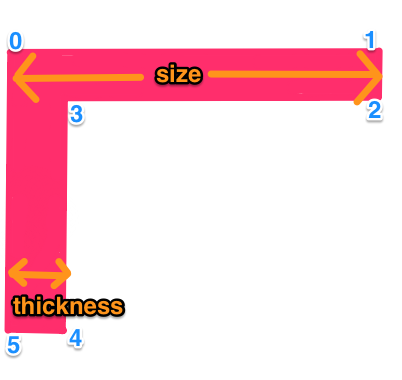
To draw the shape above by UIBezierPath
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| static CGFloat thickness = 13; static CGFloat shapeSize = 43;
CGPoint startingPoint = CGPointMake(50, 50); CGPoint points[6]; points[0] = startingPoint; points[1] = CGPointMake(points[0].x + shapeSize, points[0].y); points[2] = CGPointMake(points[0].x + shapeSize, points[0].y + thickness); points[3] = CGPointMake(points[0].x + thickness, points[0].y + thickness); points[4] = CGPointMake(points[0].x + thickness, points[0].y + shapeSize); points[5] = CGPointMake(points[0].x, points[0].y + shapeSize);
CGMutablePathRef cgPath = CGPathCreateMutable(); CGPathAddLines(cgPath, &CGAffineTransformIdentity, points, sizeof points / sizeof *points); CGPathCloseSubpath(cgPath);
UIBezierPath *path = [UIBezierPath bezierPathWithCGPath:cgPath];
CAShapeLayer *shape = [CAShapeLayer layer]; shape.path = path.CGPath; shape.fillColor = [UIColor colorWithRed:255/255.0 green:20/255.0 blue:147/255.0 alpha:1].CGColor;
[self.view.layer addSublayer:shape];
|
- Define the thickness & it’s size (see the description on that image)
- Let say your starting point is in position
{50, 50}
and you have 6 points in this shape (you can map the index with the image above)
- Convert the points to
CGPath
then add to bezier path
- Then set the bezier path to the
CAShapeLayer
and fill it with color
- Add to your
UIView
‘s layer as sublayer
Reference: